Provider Configurations
Overview
Provider configurations are the method by which you can authenticate against Terraform and OpenTofu providers through Scalr. The configurations are stored in the Scalr account, shared with environments and/or workspaces, and automatically passed to the runs. Here are a couple of key points to keep in mind when creating and managing provider configurations:
- Scalr has support for all major providers. If you don't see a provider listed, you can create a custom provider configuration.
- More than one configuration of the same type can be added to an environment.
- More than one configuration of the same type can be added to a workspace and referenced in the Terraform run by an alias.
- If the configuration is not set at the workspace level, then a provider configuration must be set as a default for an environment.
- If a configuration is set as the default for an environment, all workspaces will inherit that configuration as needed.
- The linking of provider configurations to environments is done in the provider configuration object, and setting the default for an environment is done within the environment object.
- Provider configurations cannot be deleted if they are linked to objects in use.
When provider configurations are used, do not add an empty provider configuration in the Terraform code as Scalr will automatically inject it for you.
Set Up
Provider configurations can be created through the UI or provider. In the UI, go to the account scope, expand the inventory menu, and then provider configurations. Select the type of configuration you would like to create and fill in the corresponding fields:
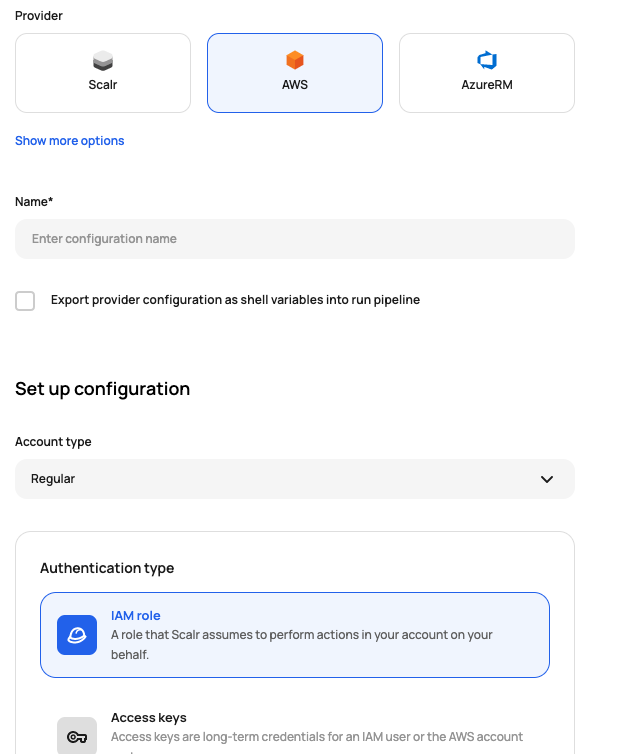
Once created, you can then share it with specific or all environments.
Here is an example using the Scalr provider to create a provider configuration:
resource "scalr_provider_configuration" "aws" {
name = "aws_service_test"
account_id = "acc-sscctbisjkl1234"
export_shell_variables = false
environments = ["*"]
aws {
account_type = "regular"
credentials_type = "role_delegation"
trusted_entity_type = "aws_service"
role_arn = "arn:aws:iam::670025224396:role/service_agent"
}
}
Now that the configuration is created, you can choose to set it as the default for an environment. To do this, navigate to the environments page in the account scope and click configuration. Then, hover over the provider and select default. If no default is selected, then the configuration must be defined at the workspace level.
Here is an example using the Scalr provider to set the default provider configuration for an environment:
resource "scalr_environment" "this" {
name = "agent_service_trust2"
account_id = "acc-sscctbisjkl1234"
default_provider_configurations = [scalr_provider_configuration.aws.id]
}
Configurations can also be assigned within the workspace scope:
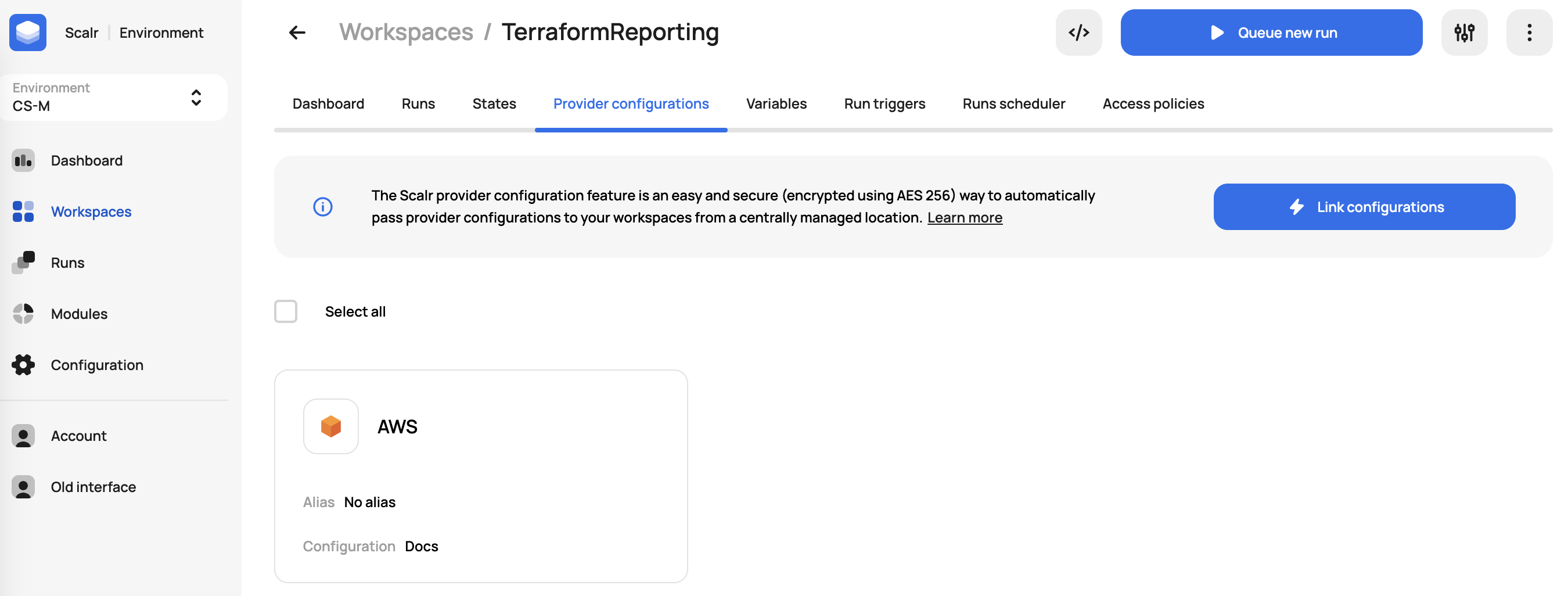
Here is an example using the Scalr provider to set the provider configuration for a workspace:
resource "scalr_workspace" "this" {
name = "this"
environment = "env-id"
provider_configuration {
id = scalr_provider_configuration.aws.id
alias = "vpc1"
}
}
If more than one configuration of the same type is assigned to a workspace, specifying an alias will pass the correct configuration during the run.
Supported Configuration Types
Scalr has support for all major Terraform providers while also allowing the usage of other providers through the "other" provider option. Please click on the provider type below for more details on usage.
Exporting Provider Configurations as Shell Variables
In the provider configurations page, you will see the option to "Export provider configurations as shell variables into the run pipeline" as an option. There are a few use cases when this option should be checked:
- If you are using the CLI of a cloud vendor in a Terraform workspace and want to authorize both the CLI and Terraform providers using a single mechanism, you can create a unified authorization approach.
- If you do not want to rely on Scalr to assume temporary credentials for provisioning infrastructure in AWS, you can create a single role that will be assumed instead. These temporary credentials can then be used to assume other hardcoded roles in the source code.
- If you have multiple provider blocks in the source code, but they require only a single credential set.
- If you only need to set default values, such as a GCP project, but want the Terraform code to decide which project resources should be provisioned.
- This must be checked if you are using the Google beta provider.
The limitation of using this option is that only one configuration can be used in the workspace.
Scalr Terraform Provider Examples
Workspaces with Multiple Configs
First, define that two provider configurations will be needed by the workspace.
resource "scalr_workspace" "this" {
name = "this"
environment = "env-id"
provider_configuration {
id = module.first.configuration_id
alias = "config1"
}
provider_configuration {
id = module.second.configuration_id
alias = "config2" # should match the provider alias defined in the Terraform configuration
}
}
Second, in your Terraform code, add the alias:
provider "aws" {
alias = "config1"
region = "us-east-1"
}
provider "aws" {
alias = "config2"
region = "us-east-1"
}
resource "aws_vpc" "example1" {
provider = "aws.config1"
cidr_block = "0.0.0.0/0"
}
resource "aws_vpc" "example2" {
provider = "aws.config2"
cidr_block = "0.0.0.0/0"
}
By defining the alias, the code will know which provider to choose when.
Multi-region with one config
It is common to want to use a single provider configuration, but have it deploy across multiple regions in the same Terraform configuration file. To do this, define the provider configuration in the workspace:
resource "scalr_workspace" "this" {
name = "this"
environment = "env-id"
provider_configuration {
id = data.scalr_provider_configuration.primary.id
}
provider_configuration {
id = data.scalr_provider_configuration.primary.id
alias = "us-west"
}
}
Then all you need to do is set the regions for the configuration:
provider "aws" {
region = "us-east-1"
}
provider "aws" {
alias = "us-west"
region = "us-west-1"
}
Supported Modules
Please see the following supported modules to help with your provider configuration setup:
Interested in improvements or adding your own module? Feel free to open a PR.
Team Ownership of Provider Configurations
For many organizations, the responsibility of creating and managing provider configurations is a shared ownership. The ownership feature allows teams to create and manager provider configurations that they own without admins having to worry about teams being able to update or delete configurations that they do not own.
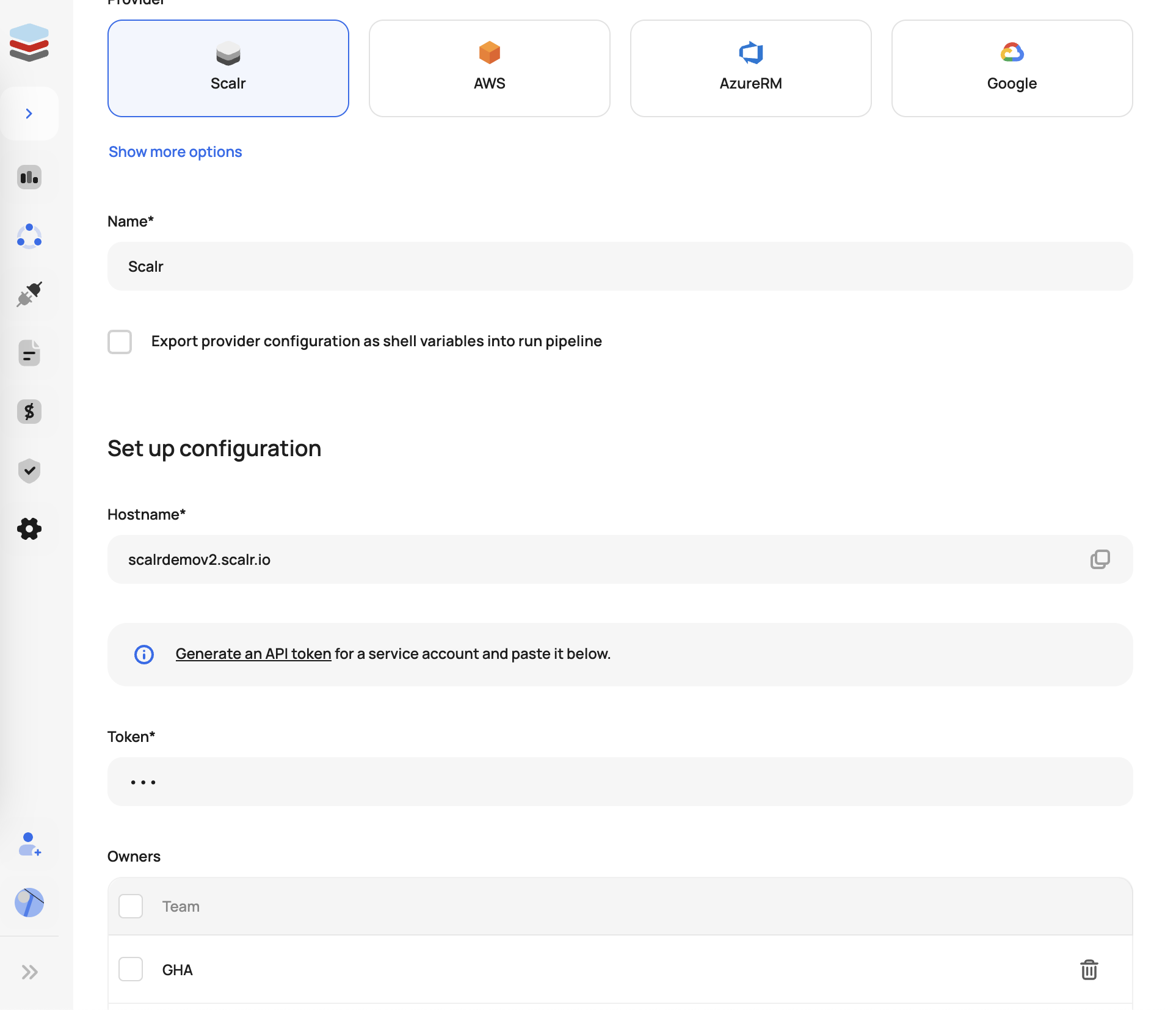
The following permissions are needed at the account scope to manage provider configurations and assign owners.
To create a configuration: cloud-credentials:create
and teams:read
.
To update a configuration: cloud-credentials:update
and teams:read
.
WARNING If a provider configuration is created without team ownership then anyone with the above permissions will be able to update, read, or delete it
Once a team creates and owns a provider configuration, they will have the option to share it with the environments they have access to.
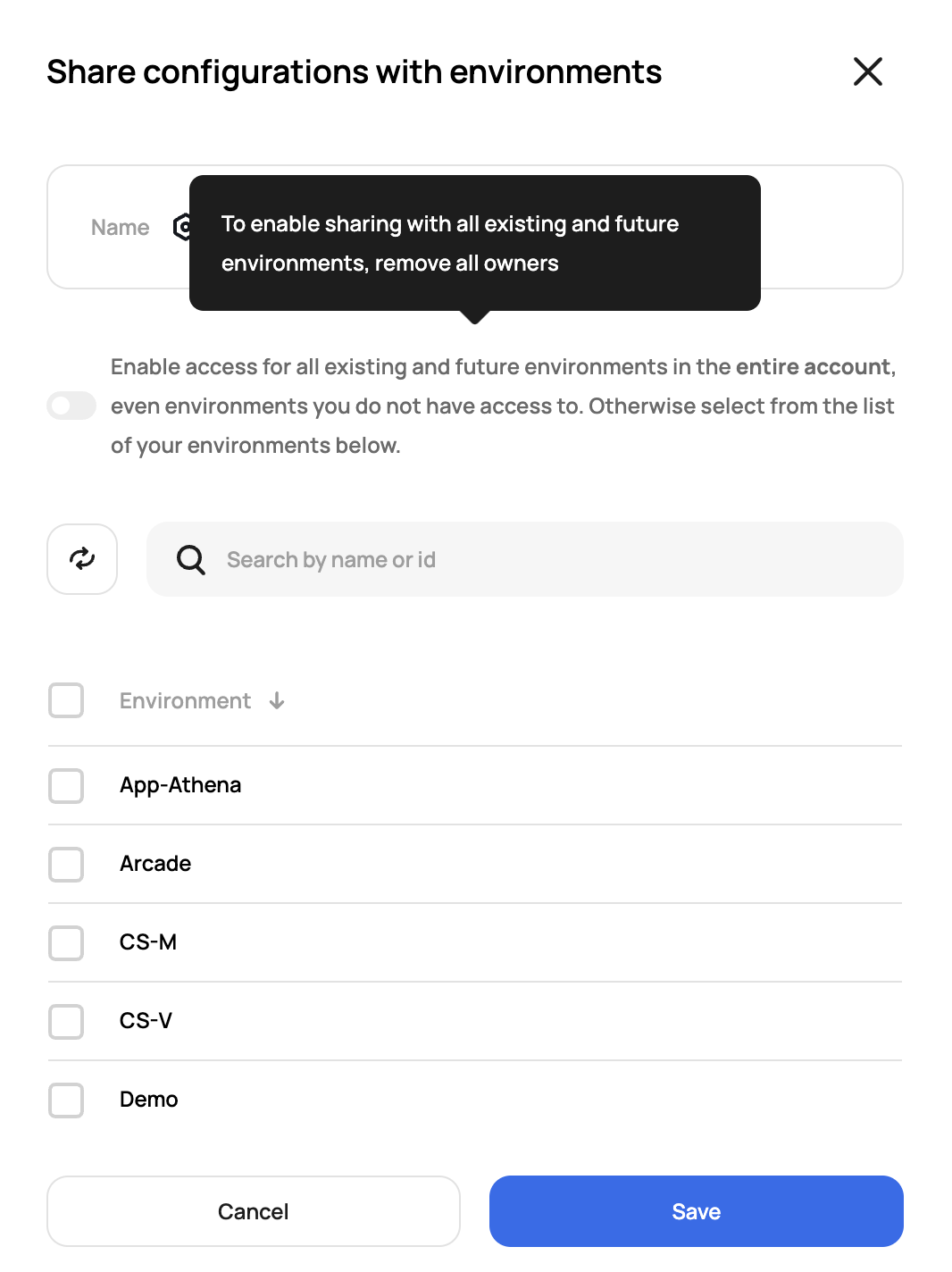
Updated 5 months ago